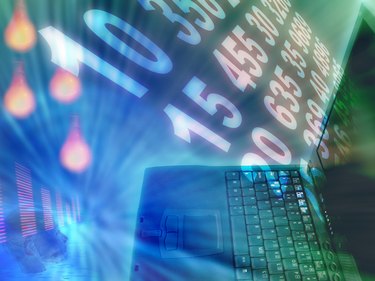
Java programs store data values in variables. When a programmer creates a variable in a Java application, he declares the type and name of the variable, then assigns a value to it. The value of a variable can be altered at subsequent points in execution using further assignment operations. The assignment statement in Java involves using the assignment operator to set the value of a variable. The exact syntax depends on the type of variable receiving a value.
Variables
Video of the Day
In Java, variables are strongly typed. This means that when you declare a variable in a Java program, you must declare its type, followed by its name. The following sample Java code demonstrates declaring two variables, one of primitive-type integer and one of an object type for a class within the application: int num; ApplicationHelper myHelp;
Video of the Day
Once a program contains a variable declaration, the kind of value assigned to the variable must be suited to the type declared. These variable declarations could be followed by assignment statements on subsequent lines. However, the assignment operation could also take place on the same line as the declaration.
Assignment
Assignment in Java is the process of giving a value to a primitive-type variable or giving an object reference to an object-type variable. The equals sign acts as assignment operator in Java, followed by the value to assign. The following sample Java code demonstrates assigning a value to a primitive-type integer variable, which has already been declared: num = 5;
The assignment operation could alternatively appear within the same line of code as the declaration of the variable, as follows: int num = 5;
The value of the variable can be altered again in subsequent processing as in this example: num++;
This code increments the variable value, adding a value of one to it.
Instantiation
When the assignment statement appears with object references, the assignment operation may also involve object instantiation. When Java code creates a new object instance of a Java class in an application, the "new" keyword causes the constructor method of the class to execute, instantiating the object. The following sample code demonstrates instantiating an object variable: myHelp = new ApplicationHelper();
This could also appear within the same line as the variable declaration as follows: ApplicationHelper myHelp = new ApplicationHelper();
When this line of code executes, the class constructor method executes, returning an instance of the class, a reference to which is stored by the variable.
Referencing
Once a variable has been declared and assigned a value, a Java program can refer to the variable in subsequent processing. For primitive-type variables, the variable name refers to a stored value. For object types, the variable refers to the location of the object instance in memory. This means that two object variables can point to the same instance, as in the following sample code: ApplicationHelper myHelp = new ApplicationHelper(); ApplicationHelper sameHelp = myHelp;
This syntax appears commonly when programs pass object references as parameters to class methods.
- Oracle: The Java Tutorials - Variables
- Oracle: The Java Tutorials - Assignment, Arithmetic, and Unary Operators
- Oracle: The Java Tutorials - Primitive Data Types
- Oracle: The Java Tutorials - Creating Objects
- Oracle: The Java Tutorials - What Is an Object?
- Oracle: The Java Tutorials - Summary of Variables
- Java Language Specification; Types, Values, and Variables; 2000
- Oracle: The Java Tutorials - Understanding Instance and Class Members