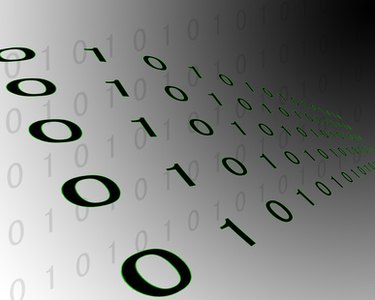
Many software products that deal with numbers and calculations have the ability to output data into a Comma Separated Value (CSV) file. This format can be an effective way of transporting data between different programs, as it is readable and fairly easy to manipulate. Many C programs that deal with data will likely have to read from a CSV file at some point.
Step 1
Consult the documentation of the program that is providing the CSV file. Determine the number of fields in each line, as well as the format of each field. For example, if a program provides a CSV with the following data: 1, "test", 34.5 You would mark down three fields: one integer, one string and one floating point number.
Video of the Day
Step 2
Create a structure containing a data member for each field identified in the CSV. Using the provided example line of 1, "test", 34.5 you would need the following structure: struct data { int col1; char *col2; float col3; };
Step 3
Create a method in your program that will handle reading the CSV file. This will need to be accessible to the rest of your program, and will likely need to work on common data structures so that other methods can access the data that has been read in. Pass the parameter by reference to remove the need for a return value. An example function prototype would be: void ParseCSV( char *filename, data& input );
Step 4
Include the standard IO header using the following code: #include <stdio.h> Add this code to the top of the source file that will be reading the CSV.
Step 5
Include the string library to enable manipulation of the CSV data using the following code: #include <string.h> Add this code to the top of the source file that will be reading the CSV.
Step 6
Create a file object, which will read in the data, using the following code: FILE * pInput;
Step 7
Create a character buffer large enough to hold one line of the file at a time. Due to constraints of the language, the most straightforward way to do this is to declare a character array of a sufficiently large size, as with: #define BUFFER_SIZE 1024
char buf[BUFFER_SIZE];
Step 8
Open the file with the following code, and assign it to your previously created FILE object: pInput = fopen("filename," "r")
Step 9
Read in a line of the file using the following code:
fgets(buf, sizeof(buf), pInput)
Step 10
Parse the CSV using the function "strtok". Create a new character string to point to the tokens, and initialize it with data from the line read in above: char *tok = strtok(buf, ",")
Step 11
Convert the received token into the appropriate data. Using the example line: 1, "test", 3.45 convert the data contained in "tok" to an integer using the following code: row.col1 = atoi(tok);
Step 12
For subsequent reads from the same line, pass "strtok" a NULL parameter instead of the buffer string you read in before: tok = strtok(NULL, ",") Then, convert the token to the appropriate data type. Using the example line 1,"test",3.45 The parsing code for a single line would be: char *tok = strtok(buf, ","); row.col1 = atoi(tok); tok = strtok(NULL, ","); row.col2 = tok; tok = strtok(NULL, ","); row.col3 = atof(tok);
Step 13
Do this for all of the entries on each line of the CSV. The function "strtok" will continue to provide the data between comma values until it runs out of data in the buffer, at which point it will return NULL. This will indicate that you have finished with the line.
Video of the Day