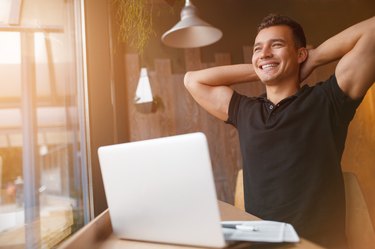
Each question in a multiple choice test consists of a question itself and several different answers, only one of which is correct. You can create a multiple choice test in Visual Basic using labels to display the questions, radio buttons for the possible answers and button controls to navigate between different questions. In the code, use arrays to save the questions and answers that the user submits and maintain a counter variable to keep track of what question the user is on. At the end of the test, mark the user's answers and display the result on the form.
Step 1
Open a new Visual Basic project. Add two labels, three radio buttons and two button controls to the form. Drag the two labels to the top with Label1 on top of Label2. Drag RadioButton1, RadioButton2 and RadioButton3 to line up vertically beneath the labels. Drag the two buttons beneath the radio buttons with Button1 on the left and Button2 on the right.
Video of the Day
Step 2
Press "F7" to open the code window. Type the following code at the class level:
Dim questions(2, 4) As String Dim answers(2) As String Dim quesNum As Integer
The first line creates a two-dimensional array. The first dimension is for each question and the second dimension is for the question itself, three answer choices and the correct answer. The second line creates an array to store the user's answers. The third line creates a counter variable that keeps track of the question the user is on.
Step 3
Type the following code:
Private Sub GetQuestions() questions = New String(,) {{"How many colors are in a rainbow?", "5", "6", "7", "7"}, {"Who starred in Pirates of the Caribbean?", "Johnny Depp", "John Malkovich", "John Cusack", "Johnny Depp"}, {"What is the capital of Florida?", "Miami", "Tallahassee", "Jacksonville", "Tallahassee"}} End Sub
This subroutine simply initializes the three questions and answers in the questions array. You can add additional questions or get them in other ways, such as through a text file, but if you do, remember to change the size of the question and answer arrays to accommodate the number of questions.
Step 4
Type the following code:
Private Sub MarkTest() Dim grade As Integer = 0 For i = 0 To 2 If answers(i) = questions(i, 4) Then grade += 1 End If Next Label1.Text = "Test finished!" Label2.Text = "You scored " & grade & " out of " & answers.Length & "!" RadioButton1.Enabled = False RadioButton2.Enabled = False RadioButton3.Enabled = False Button1.Enabled = False Button2.Enabled = False End Sub
The first line declares a subroutine that marks the test. It creates a local variable to count the score, then cycles through the answers in the questions array and the answers submitted by the user. For each answer that matches, the grade goes up by one. It then displays the score in the labels and disables the rest of the controls.
Step 5
Open the Form1_Load() subroutine and type the following code:
Me.Text = "My Multiple Choice Quiz!" GetQuestions() quesNum = 1 Label1.Text = "Question " & quesNum & " of " & answers.Length Label2.Text = questions(0, 0) Button1.Text = "Previous" Button2.Text = "Next" RadioButton1.Text = questions(0, 1) RadioButton2.Text = questions(0, 2) RadioButton3.Text = questions(0, 3)
The first line sets the title in the title bar. The next line calls the GetQuestions() subroutine. The third line initializes the question counter variable. The fourth line displays what question number the user is on. The fifth line displays question one in the label. The sixth and seventh lines change the text for the two buttons. The last three lines insert the three multiple choice answers as text for the three radio buttons.
Step 6
Open the Button1_Click() subroutine and type the following code:
If quesNum > 1 Then quesNum -= 1 Label1.Text = "Question " & quesNum & " of 3" Label2.Text = questions(quesNum - 1, 0) RadioButton1.Text = questions(quesNum - 1, 1) RadioButton2.Text = questions(quesNum - 1, 2) RadioButton3.Text = questions(quesNum - 1, 3) If Button2.Text = "Submit" Then Button2.Text = "Next" End If End If
This is the code for the "Previous" button. It first checks to see if the user pressed the button while already on the first question. If not, it decrements the question counter by one and updates the text for the labels and radio buttons to show the previous question. If the user was on the final question, the text on Button2 changes from "Submit" back to "Next."
Step 7
Open the Button2_Click() subroutine and type the following code:
If RadioButton1.Checked = True Then answers(quesNum - 1) = RadioButton1.Text ElseIf RadioButton2.Checked = True Then answers(quesNum - 1) = RadioButton2.Text ElseIf RadioButton3.Checked = True Then answers(quesNum - 1) = RadioButton3.Text End If RadioButton1.Focus() If quesNum < 3 Then quesNum += 1 Label1.Text = "Question " & quesNum & " of " & answers.Length Label2.Text = questions(quesNum - 1, 0) RadioButton1.Text = questions(quesNum - 1, 1) RadioButton2.Text = questions(quesNum - 1, 2) RadioButton3.Text = questions(quesNum - 1, 3) If quesNum = 3 Then Button2.Text = "Submit" End If Else MarkTest() End If
This is the code for the "Next" button. The first seven lines check to see what radio button the user had selected, then saves that answer to the answers array. The next line focuses the radio button selection on RadioButton1. The next line checks to see that the user is not on the final question. If this is true, it increases the question counter by one and updates the labels and radio buttons to show the next question. It then checks to see if the user is now on the final question. If so, it changes the text for the Next button from "Next" to "Submit." If the user was already on the final question and clicked "Submit," the program calls the "MarkTest" function to get the user's score.
Step 8
Save the Visual Basic program. Press "F5" to run it.
Video of the Day