Microsoft Visual Basic is a programming language based on the BASIC language, originally developed to make programming easier to learn. Visual Basic takes the familiar commands of BASIC and adds object-oriented tools and interfaces for designing WYSIWYG-like Windows applications and web controls, among many other enhancements. One relatively simple learning project for Visual Basic is the creation of a Windows calculator.
Step 1
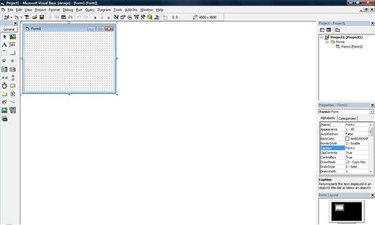
Open Visual Basic 6, and select "Standard EXE" from the new project menu. You will see a blank form appear on the screen.
Video of the Day
Step 2
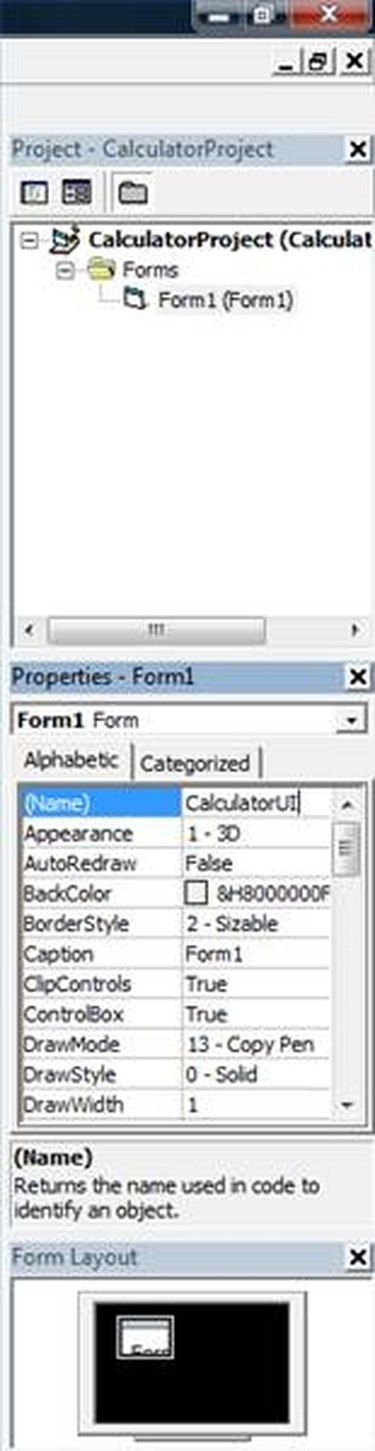
Rename your project and your form by clicking on "Project1" at the right hand side of the screen, in the project listing, and entering a new name in the "Name" line of the Properties box, which should appear below the project listing by default. Press "Enter" to accept the new name. Do the same for your form (a suggested form name is "CalculatorUI"), making sure to enter a similar name in the "Caption" property as well, which will change the text in the top bar of the form. Save the project in a new folder on your computer.
Step 3
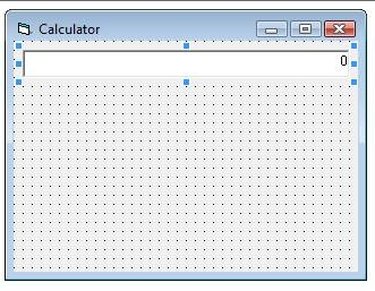
Add buttons and a text box to the form. First, add a text box, which will be where the numbers entered in the calculator appear, as well as the results of calculations. Do this by selecting the TextBox button from the toolbar at the left side of the screen, and then dragging with your mouse the size and location you desire for the TextBox. Once you've placed the TextBox you can change the size and location by dragging it to another location of the form or by dragging the handles (the small squares) along the border of the TextBox. Be sure to change the following lines in the Properties window, with the TextBox selected: "(Name)" = tbResult, "Alignment" = 1- Right Justify, "Data Format" = (click on the "..." button to select) Number, "Locked" = True, and "Text" = 0.
Step 4
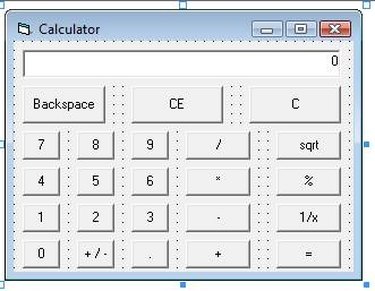
Select the CommandButton icon on the toolbar and create the first button the same way you created the TextBox to add buttons. For reference, use the Windows calculator in Standard view (Programs > Accessories > Calculator) as a basis for your calculator layout, leaving out the "MC", "MR", "MS", and "M+" buttons. On each button, change the following properties (using the "+" button as an example): "(Name)" = btnPlus, "Caption" = +. Do the same for the rest of the calculator buttons, and then save your work. Your form should now resemble the example shown here.
Step 5
Add the code. Note that if your buttons and textbox are not named the same as the code listed here expects, you will need to change the names to match your buttons and textbox, or change your buttons and textbox to match this code. First we need to create a few variables for processing calculator input:
Dim sLeft As String, sRight As String, sOperator As String Dim iLeft As Double, iRight As Double, iResult As Double Dim bLeft As Boolean
Each calculation consists of four parts: a number to the left of the operator (sLeft, iLeft), an operator (sOperator), a number to the right of the operator (sRight, iRight), and a result (iResult). In order to track whether the user is entering the left or right number, we need to create a boolean variable, bLeft. If bLeft is true, the left side of the calculation is being entered; if bLeft is false, the right side is being entered.
Step 6
Initialize the bLeft variable. We do that by creating a Form_Load subroutine, which you can either type as listed here or automatically create by double-clicking on any part of the form not covered by a button or textbox. Inside the function, we need to set bLeft to True, because the first number entered will be the left part:
Private Sub Form_Load() bLeft = True End Sub
Step 7
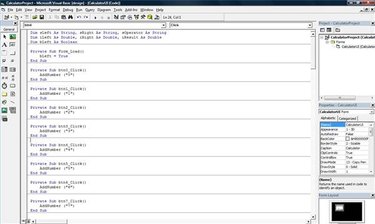
Create a subroutine that will handle the clicking of any of the number buttons. We create this as a subroutine because we use identical code for each button, and using a subroutine means not having to repeat the same code ten times. Enter the following below the Form_Load subroutine's End Sub line:
Private Sub AddNumber(sNumber As String) If bLeft Then sLeft = sLeft + sNumber tbResult.Text = sLeft Else sRight = sRight + sNumber tbResult.Text = sRight End If End Sub
As you can see, this function takes a string parameter, sNumber, which will contain the number the user has clicked on. If bLeft is true, this number is appended to the string that represents the number being entered, sLeft, and the textbox, tbResult, is updated to display the new number. If bLeft is false, the same operation is performed using sRight instead.
Finally, create a Click event function for each number that calls our AddNumber subroutine. You can do this easily by double-clicking each number button, which will create the subroutine structure for you. Then add the call to AddNumber, replacing the number in quotes with the number associated with the button. For the zero button, your code will look like this:
Private Sub btn0_Click() AddNumber ("0") End Sub
Likewise, for the one button, your code will look like this:
Private Sub btn1_Click() AddNumber ("1") End Sub
Step 8
Handle the operators: plus, minus, times, and divide. We will do this like last step, creating a subroutine that is called in the Click events for the operator buttons. The subroutine will look like the following:
Private Sub AddOperator(sNewOperator As String) If bLeft Then sOperator = sNewOperator bLeft = False Else btnEquals_Click sOperator = sNewOperator sRight = "" bLeft = False End If End Sub
If bLeft is true, meaning the user has just entered the left part of the calculation, this subroutine sets the sOperator variable we created in step 5 to equal the operator entered, which is passed to AddOperator as the string sNewOperator. The second step is to set bLeft to False, because the entry of an operator means the user is done entering the left side of the equation. In order to handle entries that string multiple operators together, such as 9 * 3 * 2 * 6, we need to also check whether bLeft is false, meaning the user has entered an operator where we were expecting an equals. First we call the Click event for the equals button (described in the next step), which does the calculation and sets tbResult to the result of what has already been entered. Then we clear sRight so the user can enter the next number, and set bLeft to False so the program knows we are entering the right hand side of the calculation next.
Finally, add an AddOperator call to the Click event of each operator button, using the same method as we used in step 7 to create the Click events for the number buttons. Your code for the plus button will look like this:
Private Sub btnPlus_Click() AddOperator ("+") End Sub
Likewise, the code for the minus button will look like this:
Private Sub btnMinus_Click() AddOperator ("-") End Sub
Step 9
Create the Click event for the equals button, which is the most complex code in this program. Create the subroutine structure like you did for the other buttons, by double-clicking the equals button on your form. Your subroutine will look like this when you've entered the code:
Private Sub btnEquals_Click() If sLeft <> "" And sRight = "" And sOperator <> "" Then sRight = sLeft End If
End Sub
The first three lines of code check to see if both sides of the calculation have been entered along with an operator. If only the left side and an operator are entered, the value of the left side is copied to the right, so we can mimic the standard calculator behavior for handling an entry like 9 * =, which multiplies 9 by itself to get a result of 81. The rest of the code will run only if left, right, and operator are entered, and starts out by copying the strings of numbers into our iLeft and iRight Double-typed variables, which can do the actual calculations. The Select Case statement allows us to run different code depending on which operator was entered, and performs the actual calculation, placing the result in iResult. Finally, we update the textbox with the result, copy the result into sLeft, reset sRight, and set bLeft = True. These last lines allow us to take the result of the calculation and use it to perform another calculation.
Step 10
Handle the last three operation buttons: sqrt, %, and 1/x. For the Click event of the square root button, your code will look like this:
Private Sub btnSqrt_Click() If sLeft <> "" Then iLeft = sLeft Else iLeft = 0 End If
End Sub
The first 11 lines of code make sure that if we don't have a value entered for either side of the equation, we substitute zero instead of trying to copy an empty string into iLeft or iRight, which will generate an error. The middle lines perform the square root function on the current part of the calculation, either left or right. Finally, we reverse the checks we did in the beginning so that a zero is copied as an empty string back into sLeft and sRight.
For the percent button, the code is similar, with one exception: the percent operation can only be performed if both left and right sides are entered.
Private Sub btnPercent_Click() If Not bLeft Then If sRight <> "" Then iRight = sRight Else iRight = 0 End If
End Sub
Lastly, the 1/x, or fraction, Click event, which is very similar to the code above:
Private Sub btnFraction_Click() If sLeft <> "" Then iLeft = sLeft Else iLeft = 0 End If
End Sub
Step 11
Add code to handle the C and CE buttons. C clears all input to the calculator, whereas CE only clears the number currently being entered.
Private Sub btnC_Click() sLeft = "" sRight = "" sOperator = "" tbResult.Text = "0" bLeft = True End Sub
Private Sub btnCE_Click() If bLeft Then sLeft = "" Else sRight = "" End If tbResult.Text = "0" End Sub
Step 12
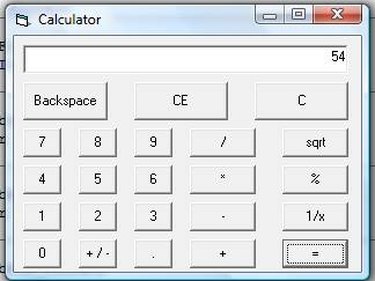
Run your calculator program and do any calculation you wish. This calculator can be easily expanded to handle more operations, more complex calculations or even to be a scientific calculator with a little extra work.
Video of the Day