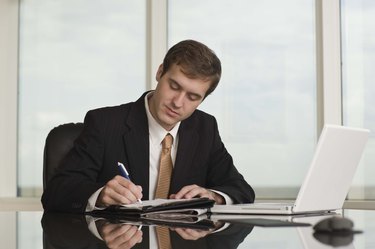
Many computer languages share common tasks such as iterating through loops, branching based on if-then-else logic and performing calculations. You can use these and other operations to construct a pseudocode program that does not run on a computer, but instead runs in your mind as you review it on paper. By brainstorming a process, identifying high-level processes and writing them down logically, you can use what you come up with to create quality software, using real programming languages.
Step 1
Think about what it takes to make a calculator work at a high level and write down those high-tasks on a sheet of paper. They might appear as shown below:
Video of the Day
inputData = Read_Input() result = Perform_Calculations(inputData) Display_Results(result)
These are the actions that occur when you type numbers into a calculator, press a function key and view the results. The first statement calls a method that reads input from a user. That method returns results which go into the Input_Data variable. The next statement passes that data to a function named Perform_Calculations. Perform_Calculations calculates a result and returns it to this code which stores it in the result variable. The final statement passes that result to a function that displays it.
Step 2
Draw a large box around those statements. This box represents the program's main module. Draw another large box on the paper and write "Read_Input()" at the top of it. This box represents the function that reads the input needed to perform calculations. Type statements into the box that you think need to occur to retrieve information from a user. Those statements might look like the ones shown below:
Print "Enter First Number" firstNumber = Read_Input Print "Enter Second Number" secondNumber = Read_Input Print "Enter an Operator" Print "Enter an Operator" operator = Read_Input Return firstNumber, secondNumber, operator
The firstNumber, secondNumber and operator variables will contain the values a user enters via some method. The final statement returns the variables to the calling module.
Step 3
Draw a second box on the paper and write "Perform_Calculations(firstNumber, secondNumber, operator) at the top of it. Add statements to this box needed to perform calculations using the two numbers and operator passed to the function. Those statements might look similar to the following statements:
if operator = "+" result = firstNumber + secondNumber
else if operator = "-" result = firstNumber - secondNumber
else if operator = "*" result = firstNumber * secondNumber
else if operator = "/" result = firstNumber / secondNumber
return result
This code uses an if statement to determine the statement to execute based on the operator's value. After one of the statements computes the result, the last statement passes the result back to the calling module.
Step 4
Draw a final box on the paper and write "Display_Result(result)" at the top of the box. Write down the statements needed to present the result to a user, such as this:
Print result
Review the pseudocode and follow the logic beginning at the first statement in the main module. Look for logical flaws in the code as you step through it. Identify those if they exist, and correct your pseudocode if necessary.
Video of the Day