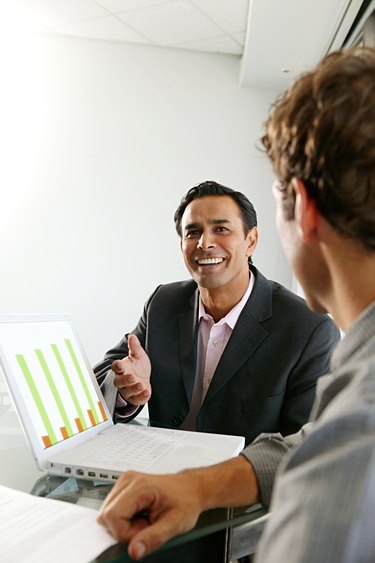
Histograms are commonly found as a chart option in analyzing data in spreadsheet software, and in image editing software for showing the distribution of tones from black to white in an image. In the C programming language, using an array to hold the frequency count simplifies creating a histogram of your data set. While data sets used for creating histograms usually contain integer values, characters and even strings can be counted and graphed.
Step 1
Populate an integer array called "values" with your data set, and set the "numvalues" variable to the number of values in your data set. These could be randomly generated, read in from a file, or interactively collected from the user. This example initializes these variables when they are declared:
Video of the Day
int numvalues = 20; int values[numvalues] = { -3, 2, -2, 4, 5, 4, 2, 5, 4, 5, -1, 2, 3, 4, 7, 4, 2, 0, 7, -3 };
Step 2
Set up two integer variables (i and j) to use as iterators:
int i = 0, j = 0;
Step 3
Iterate through your data -- the values array -- and set the "maxval" variable to the maximum value of your data:
int maxval = 0; for (i=0; i<numvalues; i++) { if (values[i] > maxval) maxval = values[i] }
Step 4
Step through your data and set the "minval" variable to the maximum value of your data:
int minval = maxval; for (i=0; i<numvalues; i++) { if (values[i] < minval) minval = values[i] }
Step 5
Declare a variable "freqsize" to hold the size of your frequency array:
int freqsize = maxval - minval + 1 ;
Step 6
Declare an array to hold the frequency counts and initialize each array element to zero:
int frequency[freqsize]; for (i=0; i<freqsize; i++) { frequency[i] = 0; }
There is one array element for each possible value in your data set.
Step 7
Step through each value in your data set, adding one to the frequency array element corresponding to that value:
for (i = 0 ; i < numvalues ; i++) { int index = values[i] - minval; frequency[index]++ }
The index corresponding to the current value is generated by shifting the value by the minimum value.
Step 8
Step through each element in the frequency array. Print the current value (calculated by shifting the iterator "i" by the minimum value). Print the number of stars (*) corresponding to the frequency the current value by looping from one to the value stored in the frequency array, printing a single star each time:
for (i=1; i<=freqsize; i++) { printf("%2d\t|", i + minval); for(j=0; j<frequency[i]; j++) { printf("*") } printf("\n") }
Video of the Day